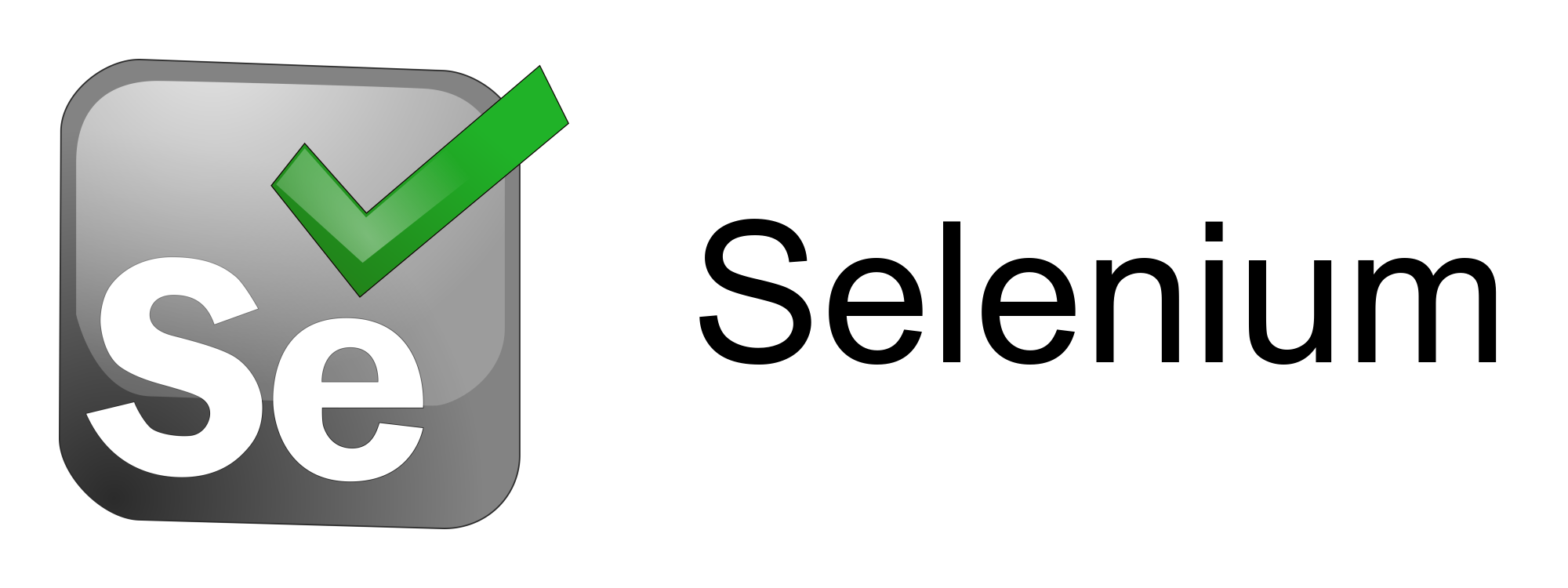
I have a project where I need to have a persistent Selenium session. There’s a script which will leave a browser window open when it exits. When the script runs again it should connect to the same window.
Derive a new class from selenium.webdriver.Remote
.
from selenium import webdriver
from selenium.webdriver.common.desired_capabilities import DesiredCapabilities
from selenium.common.exceptions import WebDriverException
SELENIUM_URL = "http://127.0.0.1:4444/wd/hub"
class PersistentRemote(webdriver.Remote):
def __init__(self, session_id=None):
super(PersistentRemote, self).__init__(
command_executor=SELENIUM_URL,
desired_capabilities=DesiredCapabilities.CHROME,
)
#
# This will create a new window.
if session_id is not None:
# Close new window.
self.close()
# Change to other session.
self.session_id = session_id
# Will raise WebDriverException if session no longer valid.
try:
self.title
except WebDriverException:
raise WebDriverException(f"Session '{session_id}' no longer valid.")
The crux of this is that a new window will always be opened. If, however, you have an existing session, then close the new window and connect to the other session.
Here’s what the client code looks like.
import pickle
# A file to store the session information.
#
SELENIUM_SESSION_PICKLE = "selenium-session.pkl"
try:
session = pickle.load(open(SELENIUM_SESSION_PICKLE, 'rb'))
browser = persistent.PersistentRemote(session)
# If you get to here then you are using an existing session.
except (FileNotFoundError, persistent.WebDriverException):
# If you end up here then you are creating a new session.
browser = persistent.PersistentRemote()
pickle.dump(browser.session_id, open(SELENIUM_SESSION_PICKLE, 'wb'))
browser.get("https://www.google.com")