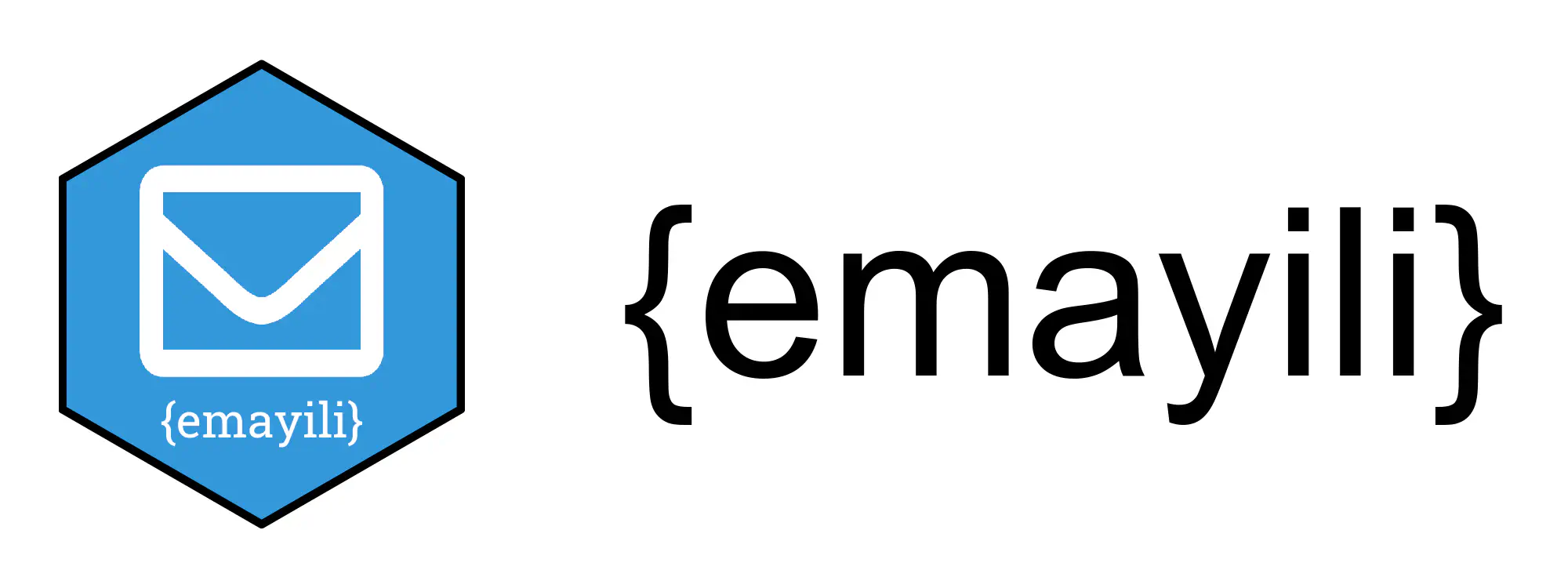
Suppose that you want to use {emayili}
to send birthday messages (this post motivated by issue #61).
Specifically, you want to
- send one message to the person having a birthday and
- send multiple messages to other people, reminding them of the birthday.
Load a couple of packages.
library(emayili)
library(dplyr)
Set up some variables to hold the various email addresses.
# Who's sending the messages?
FROM <- "micceee@gmail.com"
# Who has a birthday?
TO <- "bob@gmail.com"
# Who needs to be reminded?
OTHERS <- c("alice@gmail.com", "craig@yahoo.com", "grace@gmail.com")
SMTP_USERNAME = Sys.getenv("SMTP_USERNAME")
SMTP_PASSWORD = Sys.getenv("SMTP_PASSWORD")
SMTP_SERVER = Sys.getenv("SMTP_SERVER")
SMTP_PORT = Sys.getenv("SMTP_PORT")
Get the SMTP server details from environment variables.
smtp <- server(
host = SMTP_SERVER,
port = SMTP_PORT,
username = SMTP_USERNAME,
password = SMTP_PASSWORD
)
Message to the Birthday Boy
Now let’s create a simple message to Bob, the birthday boy.
email <- emayili::envelope() %>%
from(FROM) %>%
to(TO) %>%
subject("Happy Birthday, Bob!") %>%
attachment(path = "meme-happy-birthday-magnificent-bastard.jpg")
Such a message would be incomplete without a suitable meme.
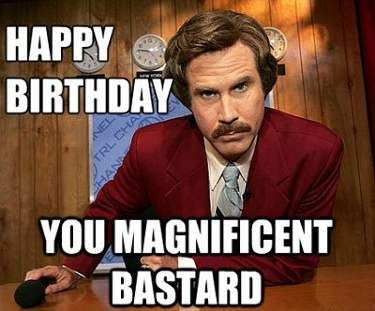
And send it via the SMTP server.
smtp(email)
If you want to see the details of the interaction with the SMTP server, include the verbose = TRUE
option.
Okay, now that we’re done with that, we’ll pause briefly. This is not strictly necessary, but it’s always good practice not to hammer any sort of service.
# Give the server a breather.
#
Sys.sleep(5)
Reminders to Friends
Now we’re going to send reminder messages to Bob’s friends.
Looping
We could to this by simply looping over the recipients’ addresses.
for (other in OTHERS) {
message("Sending mail to ", other, ".")
envelope() %>%
from(FROM) %>%
to(other) %>%
subject("It's Bob's birthday!") %>%
smtp()
}
The disadvantage to this is that there are multiple interactions with the SMTP server, so more chances for something to break. This would become especially apparent if there were many addresses in the list.
We could introduce a delay into the loop, which would probably reduce the likelihood of problems, but then this would also mean that the process takes a lot longer.
Batching
A better alternative is to send all of the messages as a single batch. Just one interaction with the SMTP server.
emayili::envelope() %>%
from(FROM) %>%
bcc(OTHERS) %>%
subject("It's Bob's birthday!") %>%
smtp()
You’d probably want to use bcc()
rather than to()
here for the added anonymity associated with Bcc
recipients.