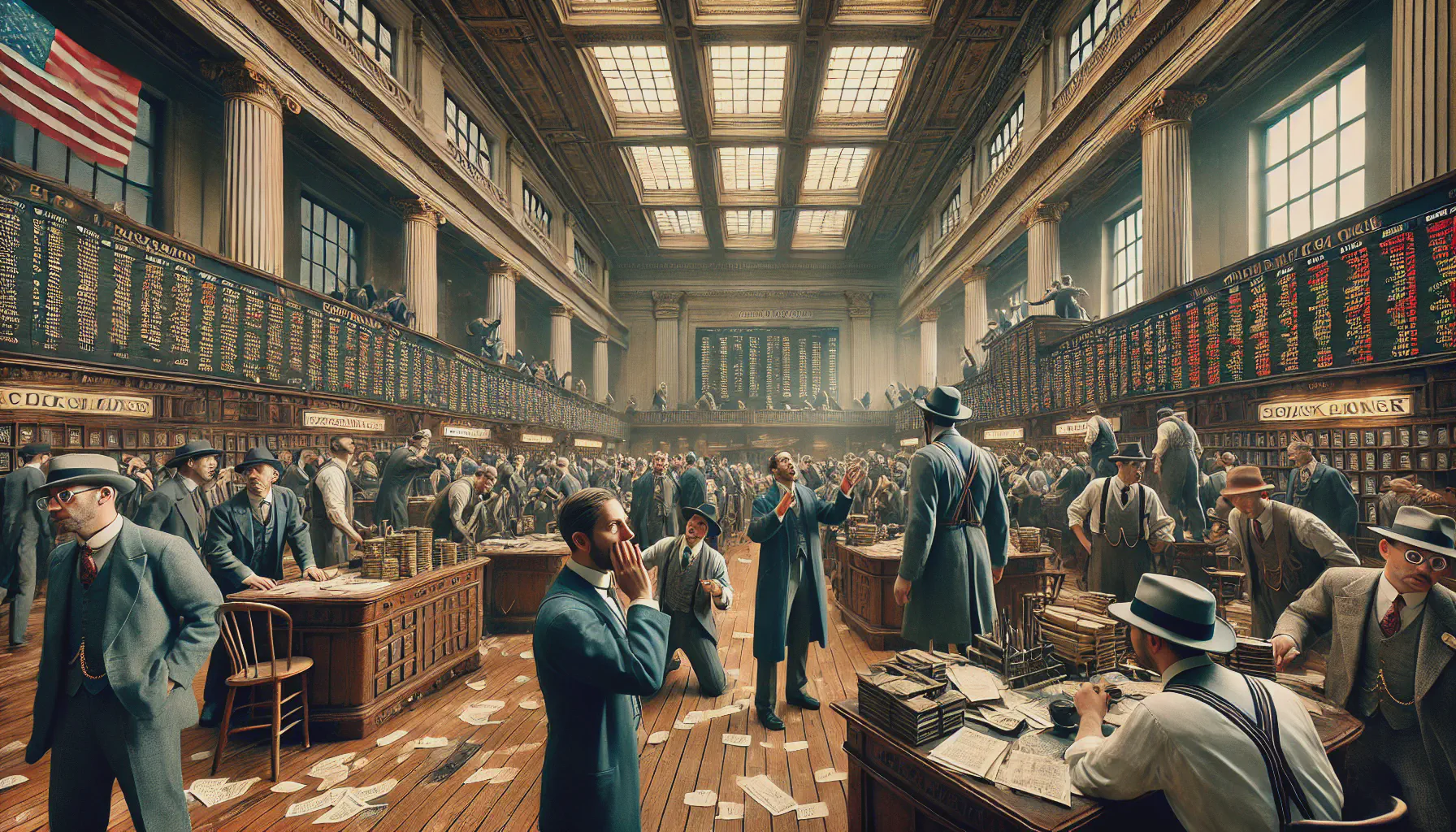
In this post I show how to connect to the Interactive Brokers API from MATLAB. The “obvious” way generates a SSL error. This is an alternative approach using the system curl
command. It’s a bit of a hack but it does the job.
Client Portal
First we’ll need to launch the client portal. Follow these instructions.
Python
Assuming that you followed the instructions you’ll have already made an API request using curl
. Let’s warm up to MATLAB by making a request using Python.
import json
import requests
URL = "https://localhost:5000/v1/api/portfolio/accounts"
headers = {
"accept": "application/json",
"Content-Type": "application/json",
"User-Agent": "Test",
}
response = requests.get(URL, headers=headers, verify=False)
print(response.status_code)
print(json.dumps(response.json(), indent=2))
The script should print a (OK
) status code and the details of the authenticated account.
If you do not set verify
to false then the GET request will raise an SSLError
exception. When you set it to true you’ll simply see a InsecureRequestWarning
. According to Interactive Brokers this is just fine. Personally I think that insisting on an SSL connection but then telling you that it’s okay to ignore SSL errors is just silly. But, hey, that’s just me. You might think that you can just connect via HTTP rather than HTTPS. You would be mistaken.
MATLAB
Native Approach
Start by trying to use the webread()
function to access the API.
URL = "https://localhost:5000/v1/api/portfolio/accounts";
HeaderFields = [
"accept", "application/json";
"Content-Type", "application/json";
"User-Agent", "Test"
];
Options = weboptions("HeaderFields", HeaderFields, "Timeout", 15, "CertificateFilename", "");
response = webread(URL, Options);
disp(response);
Sadly this will not work. You’ll almost certainly get a SSL error stating that the certificate doesn’t match the localhost
host name. Setting CertificateFilename
to an empty string was supposed to disable SSL checks but it doesn’t appear to make any difference.
Curl Hack
So, here’s the dirty hack. The curl
command has an option which will allow it to ignore SSL errors. We’ll use the system()
function to pass our request to the curl
command.
URL = "https://localhost:5000/v1/api/portfolio/accounts";
headers = "-H 'accept: application/json' -H 'Content-Type: application/json' -H 'User-Agent: Test'";
command = sprintf("/usr/bin/curl -k %s %s", headers, URL);
[status, response] = system(command);
disp(response);
data = jsondecode(response);
disp(data);
If you get an empty response from the API it probably indicates that your login session has lapsed.
You probably don’t need to redirect stderr
but I found this to be helpful because of an annoying warning about libcurl
that cropped up on one platform.
Self-Signed Certificate
Another option, which I have not yet had the time or motivation to try, is to create a self-signed certificate. This is the rough outline of the process:
- Use
openssl
to generate a self-signed certificate. The certifcate will be in PEM format and there will be four files:.crt
,.csr
,.key
and.pem
. - In MATLAB use
weboptions()
to specify the path to the.crt
file. - You also need to update the Client Portal to use this certificate.
- Convert the certificate into JKS format.
- When converting you will need to provide a password.
- Update
root/conf.yaml
to reference the.jks
file and password.
For the moment the dirty hack will suffice. If things get more serious then I’ll explore this option. No point in shaving this particular yak just yet.