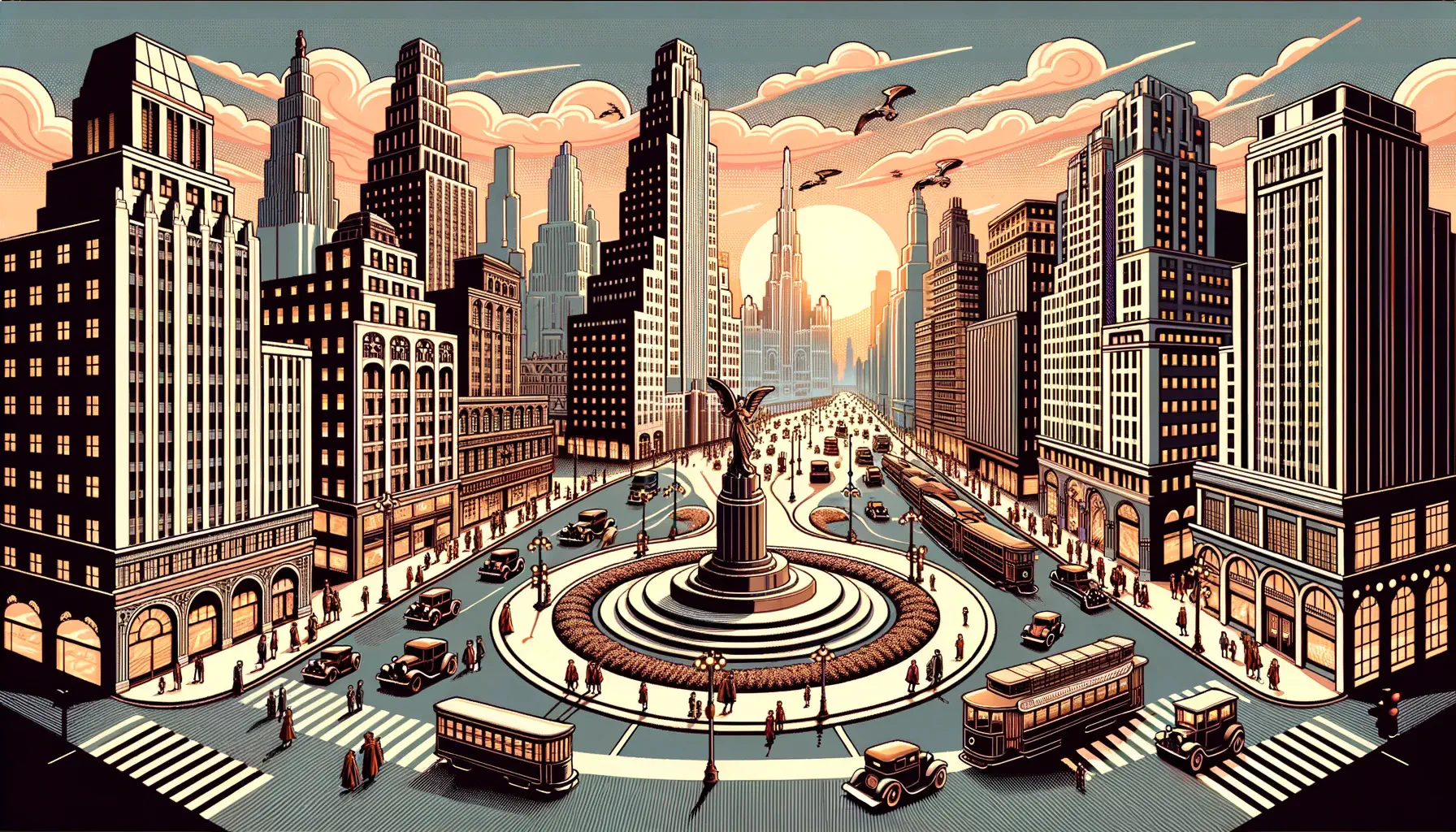
Redirects instruct web browsers to automatically reroute from one URL to another. They are especially vital when website structures change, pages get deleted, or content moves to a new location. Whether you’re rebranding, restructuring, or simply optimizing your site’s user experience, Gatsby offers powerful tools for handling redirects seamlessly. In this post, we’ll delve into the intricacies of implementing and managing redirects with Gatsby, ensuring your visitors always land in the right place.
🚀 TL;DR See links below.
Situation
We will build on the site created in a previous post. The site at present has the following paths:
/
(the landing page)/what-is-asciidoc/
/what-is-gatsby/
and/what-is-tailwind/
.
Suppose that in an earlier version of the site these pages were found at the following paths:
/
/asciidoc/
/gatsby/
/tailwind/
.
Now there might be links from other pages which refer to these paths. We would not want to lose all of that inbound link goodness, but at the same time, if somebody followed those links they would lead to a 404 (not found) error. Redirects are an elegant solution to this problem. We would simply redirect each of the old paths to the corresponding new path as follows:
/asciidoc/
→/what-is-asciidoc/
/gatsby/
→/what-is-gatsby/
/tailwind/
→/what-is-tailwind/
.
Redirects in Code
The createRedirect()
function is used to add a redirect to a Gatsby site. The first (and simplest) option is to add individual redirects in code.
Add the following to the createPages()
function in gatsby-node.js
:
createRedirect({
fromPath: "/asciidoc/",
toPath: "/what-is-asciidoc/",
})
createRedirect({
fromPath: "/gatsby/",
toPath: "/what-is-gatsby/",
})
createRedirect({
fromPath: "/tailwind/",
toPath: "/what-is-tailwind/",
})
Now if you visit any of the old paths you will automatically be redirects to the new path.
🚀 TL;DR
Show me the code. Look at the 6-redirect-code
branch. This version of the site is deployed here.
Redirects from File
For a large site with numerous redirects implementing each in code is going to be laborious to set up and painful to maintain.
The solution to this is to list all of the redirects in a separate file and then simply iterate over them in code.
First create a redirects.json
file with the followings contents:
[
{
"fromPath": "/asciidoc/",
"toPath": "/what-is-asciidoc/"
},
{
"fromPath": "/gatsby/",
"toPath": "/what-is-gatsby/"
},
{
"fromPath": "/tailwind/",
"toPath": "/what-is-tailwind/"
}
]
Then update the createPages()
function in gatsby-node.js
to include that file and loop over the contents:
redirects.forEach(redirect => createRedirect(redirect))
🚀 TL;DR
Show me the code. Look at the 7-redirect-file
branch. This version of the site is deployed here.
Splat Redirects
You might have noticed that the redirects all follow the same pattern. We can take advantage of this regularity to simplify the redirect configuration by using splat redirects.
Now the three explicit redirects can be replaced by a single pattern redirect:
[
{
"fromPath": "/*/",
"toPath": "/what-is-*/"
}
]
You can freely mix explicit and splat redirects.
🚀 TL;DR
Show me the code. Look at the 8-redirect-splat
branch. This version of the site is deployed here.
How Does it Work?
Since Gatsby is simply a static site generator it has no way to handle redirects. These would normally be handled either (1) by the server or (2) on the client. So how does Gatsby convey the information about the redirects to the server? This depends on the platform.
Gatsby Cloud
If you are deploying onto Gatsby Cloud then during the build process a _redirects.json
file will be created in the public/
folder. This file contains all of the redirect details.
{
"redirects": [
{
"fromPath": "/asciidoc/",
"isPermanent": false,
"ignoreCase": true,
"redirectInBrowser": false,
"toPath": "/what-is-asciidoc/"
},
{
"fromPath": "/gatsby/",
"isPermanent": false,
"ignoreCase": true,
"redirectInBrowser": false,
"toPath": "/what-is-gatsby/"
},
{
"fromPath": "/tailwind/",
"isPermanent": false,
"ignoreCase": true,
"redirectInBrowser": false,
"toPath": "/what-is-tailwind/"
}
]
}
Netlify
When deploying onto Netlify a _redirects
file will be created in the public/
folder.
/asciidoc/ /what-is-asciidoc/ 302
/gatsby/ /what-is-gatsby/ 302
/tailwind/ /what-is-tailwind/ 302
You can also add a _redirects
file in the static/
folder and its contents will be added before these entries.
Conclusion
Ensuring a seamless user experience is crucial in web development, and redirects play an indispensable role. Gatsby equips developers with robust tools to handle redirects, whether they’re singular changes or pattern-based. By leveraging these capabilities, not only can you maintain the integrity of inbound links and avoid 404 errors, but you can also provide a fluid journey for your site visitors. So, the next time you find yourself restructuring your site or revising your URLs, remember the power and simplicity of Gatsby’s redirect mechanisms.
More information on redirects in Gatsby can be found in the official documentation.