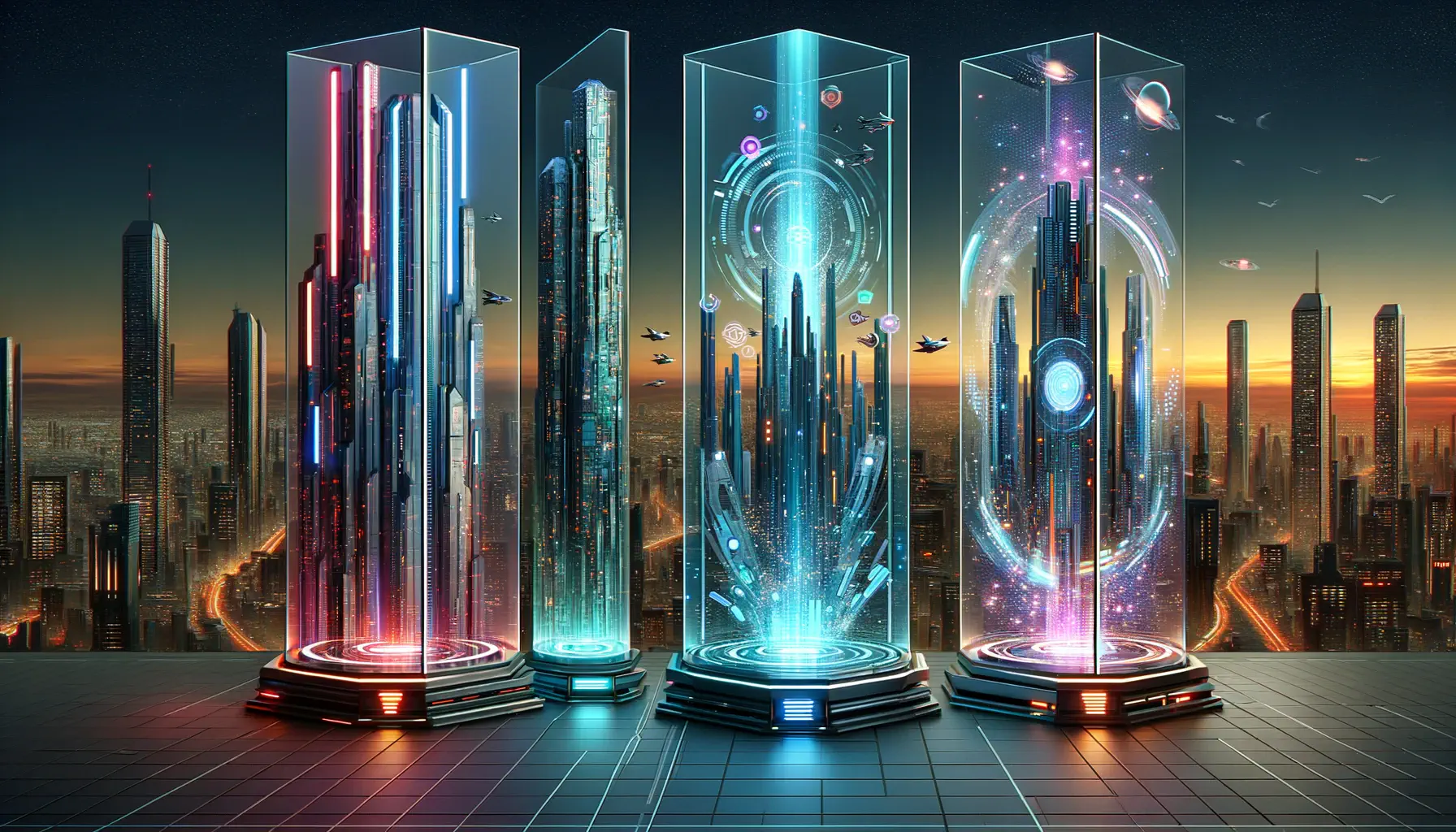
Want to use Tailwind CSS with your Next.js site? Here’s how to get that set up. Also how to wrap the whole project in a Docker image.
🚀 TL;DR The code for this project can be found here.
Create a Next.js Project
Create a new project. Accept all of the default responses (most importantly accept the option to use Tailwind).
npx create-next-app@latest next-tailwind
Change to the newly created directory.
cd next-tailwind/
Install Packages
Now install some packages, most notably tailwindcss
.
npm install tailwindcss postcss autoprefixer
Initialise & Configure Tailwind
Next we need to initialiase Tailwind.
npx tailwindcss init -p
That will add two new files: tailwind.config.js
and postcss.config.js
. Edit tailwind.config.js
.
/** @type {import('tailwindcss').Config} */
module.exports = {
purge: ['./pages/**/*.{js,ts,jsx,tsx}', './components/**/*.{js,ts,jsx,tsx}'],
darkMode: false,
theme: {
extend: {},
},
variants: {
extend: {},
},
plugins: [],
};
That tells Tailwind which files it needs to monitor for CSS directives.
Now create styles/globals.css
. This will include the basic Tailwind components. You can also add any custom CSS in here.
@tailwind base;
@tailwind components;
@tailwind utilities;
Add Content
Now we need to add a page to the site. Edit app/page.tsx
. This file should contain some boilerplate content. Replace that with the following.
import Image from "next/image";
export default function Home() {
return (
<div className="flex justify-center items-center h-screen">
<h1 className="text-4xl font-bold">
Hello, Next.js with Tailwind!
</h1>
</div>
)
}
Test on Host
Launch the app directly on your host machine.
npm run dev
Visit the site at http://127.0.0.1:3000/. If you look in the <head>
of the document (you’ll need to use Developer Tools in your browser to do this) you’ll see a link to a dynamically generated CSS file.
Dockerise
Cool. Next we’ll wrap this all up in a Docker image. Here’s the Dockerfile
.
FROM node:alpine
WORKDIR /usr/src/app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
CMD ["npm", "start"]
Build the Docker image.
docker build -t next-tailwind .
And finally create a running container.
docker run --rm -p 3000:3000 next-tailwind
Head back to http://127.0.0.1:3000/ to see the site now served from the Docker container.
🐳 See this for a similar approach using Gatsby.