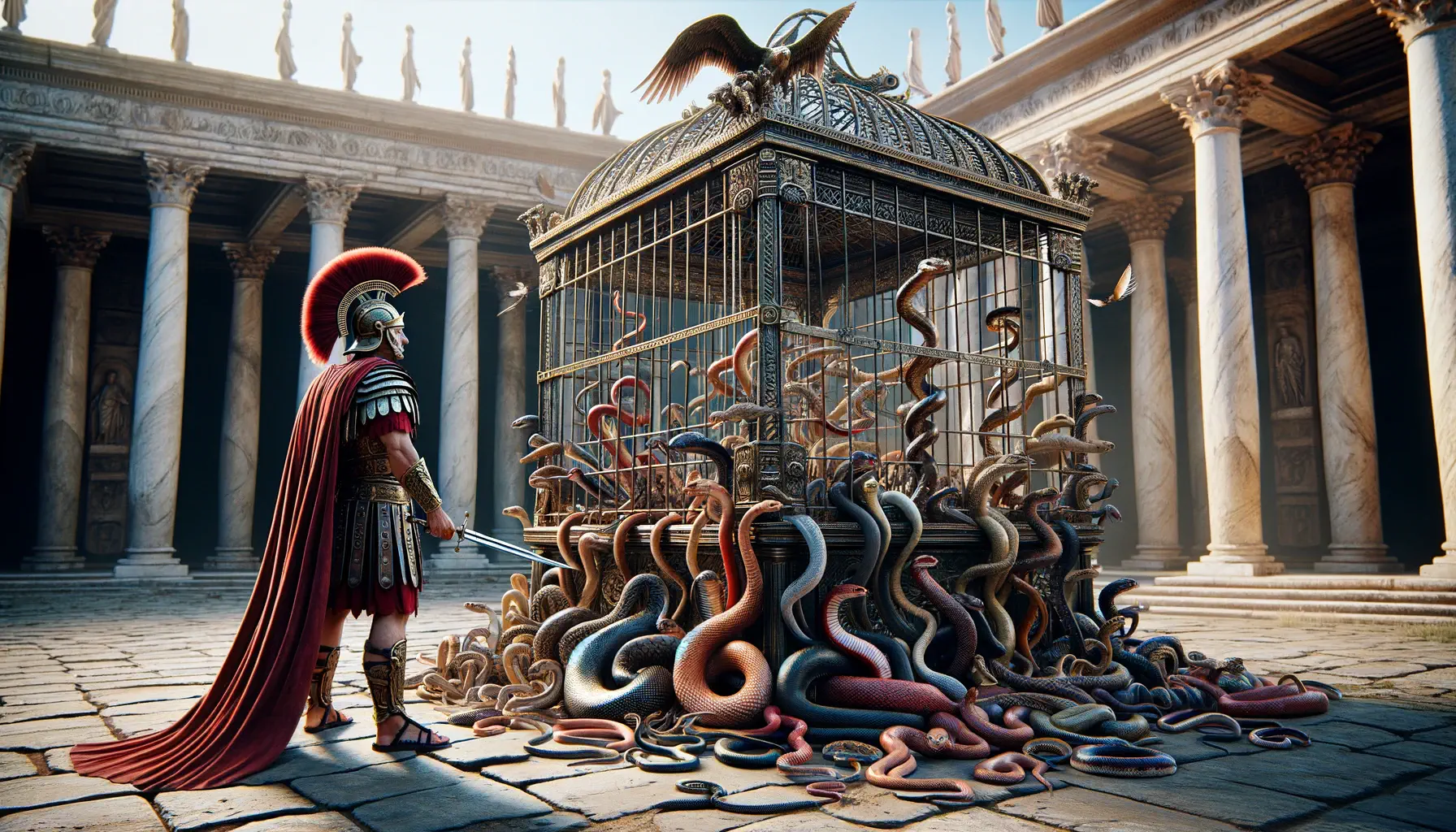
Is my code secure? This is something that we should all be thinking (if not worrying) about. A thorough security audit would be the ideal, but what if you don’t have the skills or resources for that? Well, there are some tools that will at least get you part way there.
In a previous post we looked at how pre-commit hooks can be used to check and update stylistic problems in your Python code. We can do something similar for security.
If you don’t yet have pre-commit
set up for your project, take a quick look at this first.
Bandit
Bandit is a tool for finding common security vulnerabilities in Python code. Bandit constructs an Abstract Syntax Tree (AST) for each Python file then checks those for issues. Once it has analysed all files it generates a report. Check out the Bandit documentation for more details.
Add Bandit to your .pre-commit-config.yaml
.
- repo: https://github.com/PyCQA/bandit
rev: 1.7.6
hooks:
- id: bandit
args: ["-r", "."]
Create a .bandit
file to specify Bandit configuration. For example, below we ignore both the .venv/
and tests/
sub-directories.
[bandit]
exclude = ./.venv,./tests
skips = B104
If you want to run Bandit over all of the files in the repository immediately then do this:
pre-commit run --all-files
Otherwise it’ll be run over modified files on commit.