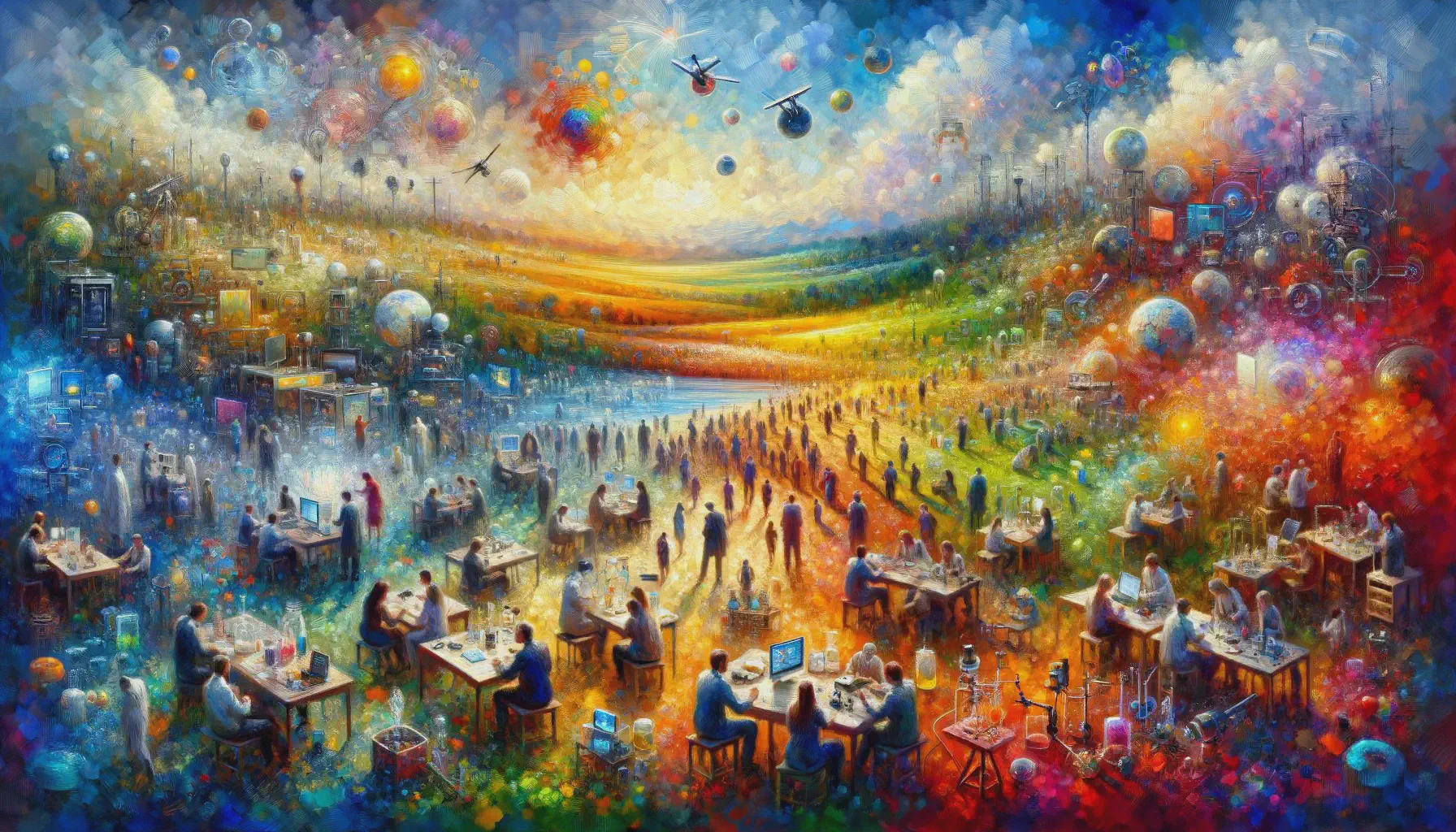
There are many tools for generating CSS selectors and XPath expressions. However, short of using them in your code, how can you quick test them? In this post I’ll show how you can use your browser’s Developer Tools to establish that your CSS or XPath is doing what you intend.
Target Site
For the purpose of illustration we’ll use https://franklin.genoox.com/clinical-db/home as the target site. We’ll aim to select the search input field.
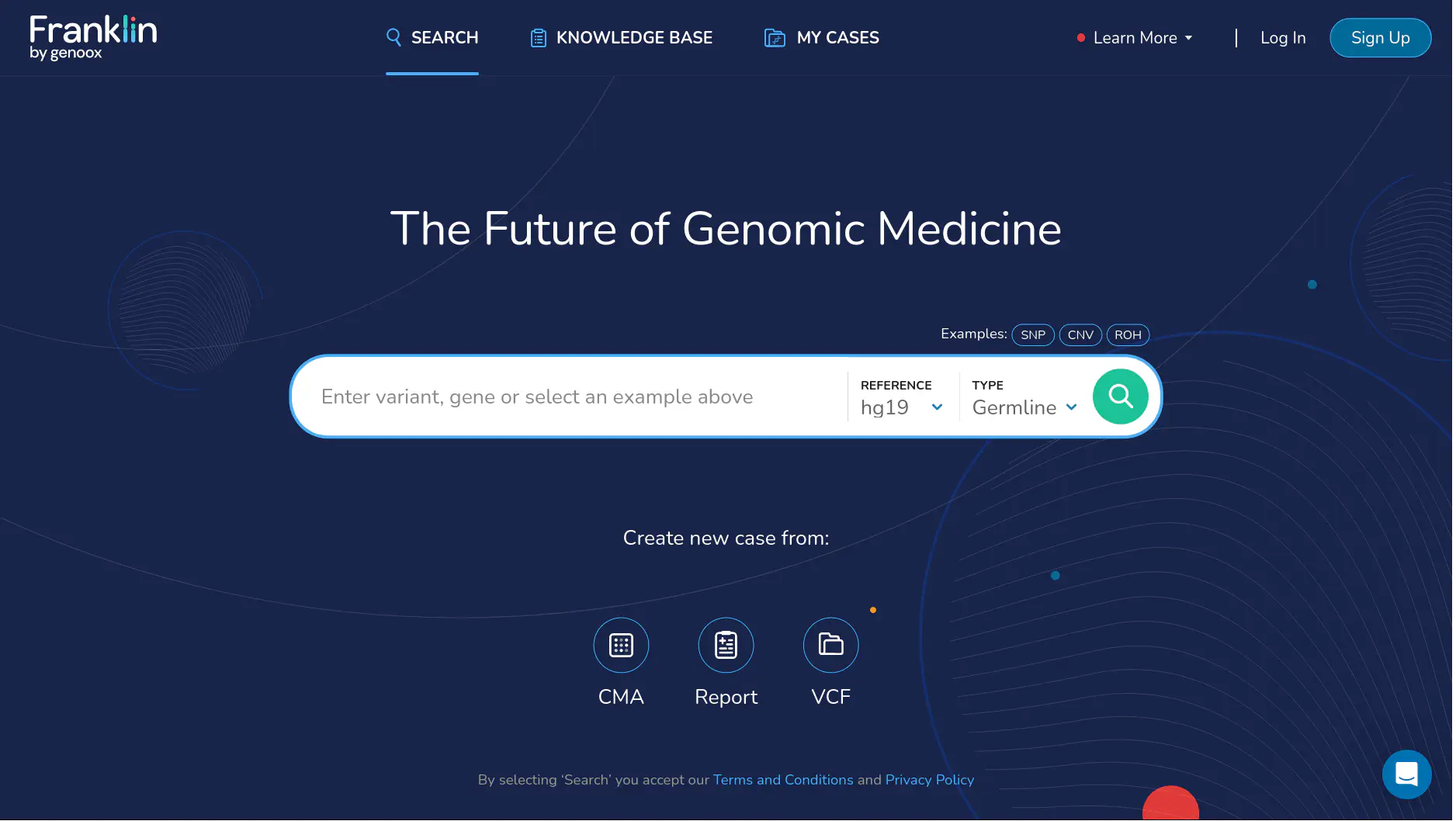
CSS
By inspection of the page HTML I determined that .search-wrapper > input
was the appropriate CSS selector.
To verify the selector, open the page in a browser and the inspect the page with Developer Tools. Change to the Console tab.
css = ".search-wrapper > input";
document.querySelectorAll(css);
The result should be a NodeList
object and the first (and only) element in the list will be the required <input>
tag.
XPath
It’s a simple matter to translate the CSS selector into XPath //*[@class='search-wrapper']/input
.
Testing this is a little more work. Same process as before though. Run the following in the Developer Tools Console.
xpath = "//*[@class='search-wrapper']/input";
result = document.evaluate(xpath, document, null, XPathResult.ORDERED_NODE_SNAPSHOT_TYPE, null);
for (var i = 0; i < result.snapshotLength; i++) {
console.log(result.snapshotItem(i));
}
Again the required <input>
tag should be listed.
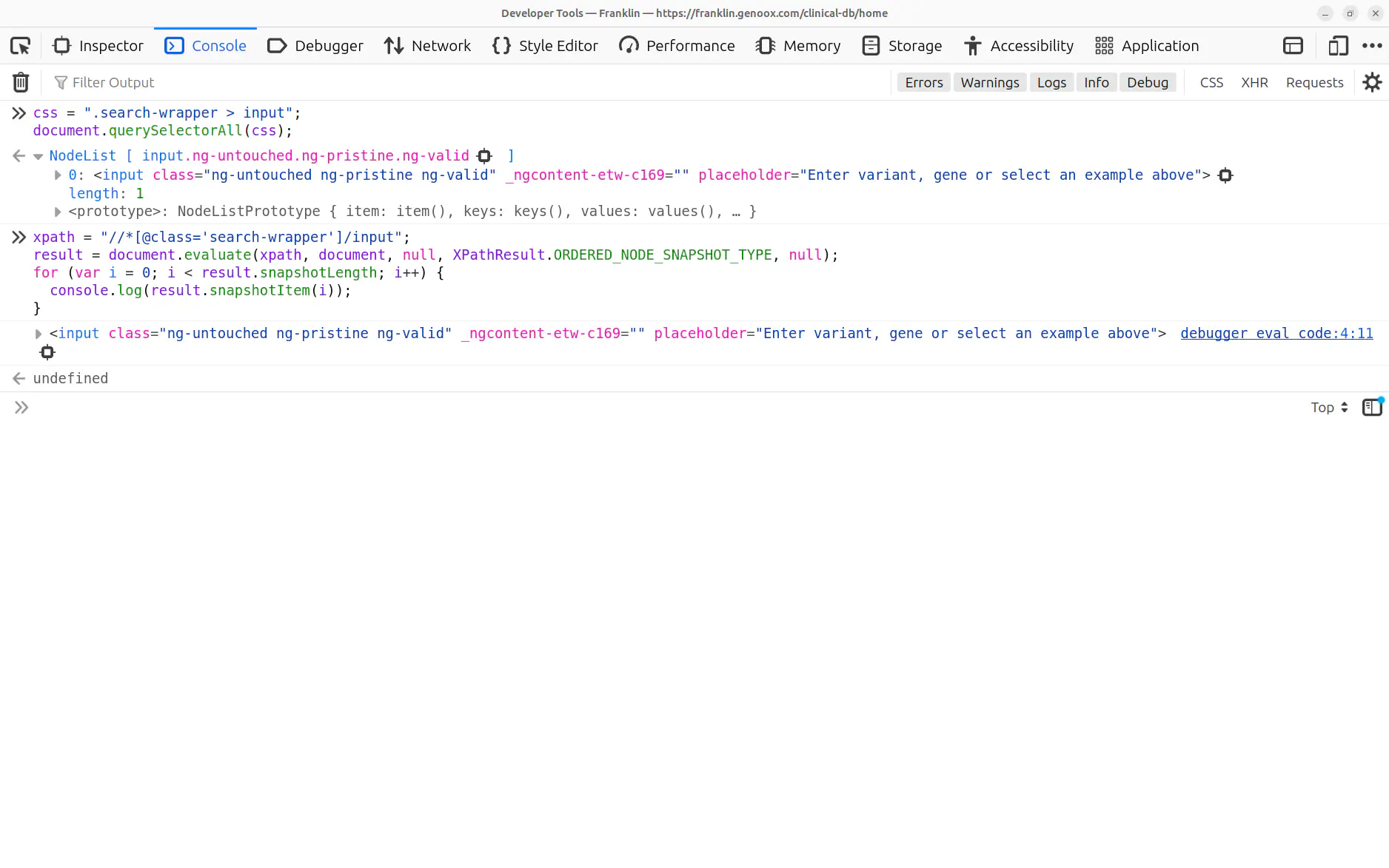
Conclusion
While perhaps not the most aesthetic way to test, these approaches allow you to reliably establish whether your CSS selectors and XPath expressions are doing what they are supposed to do!