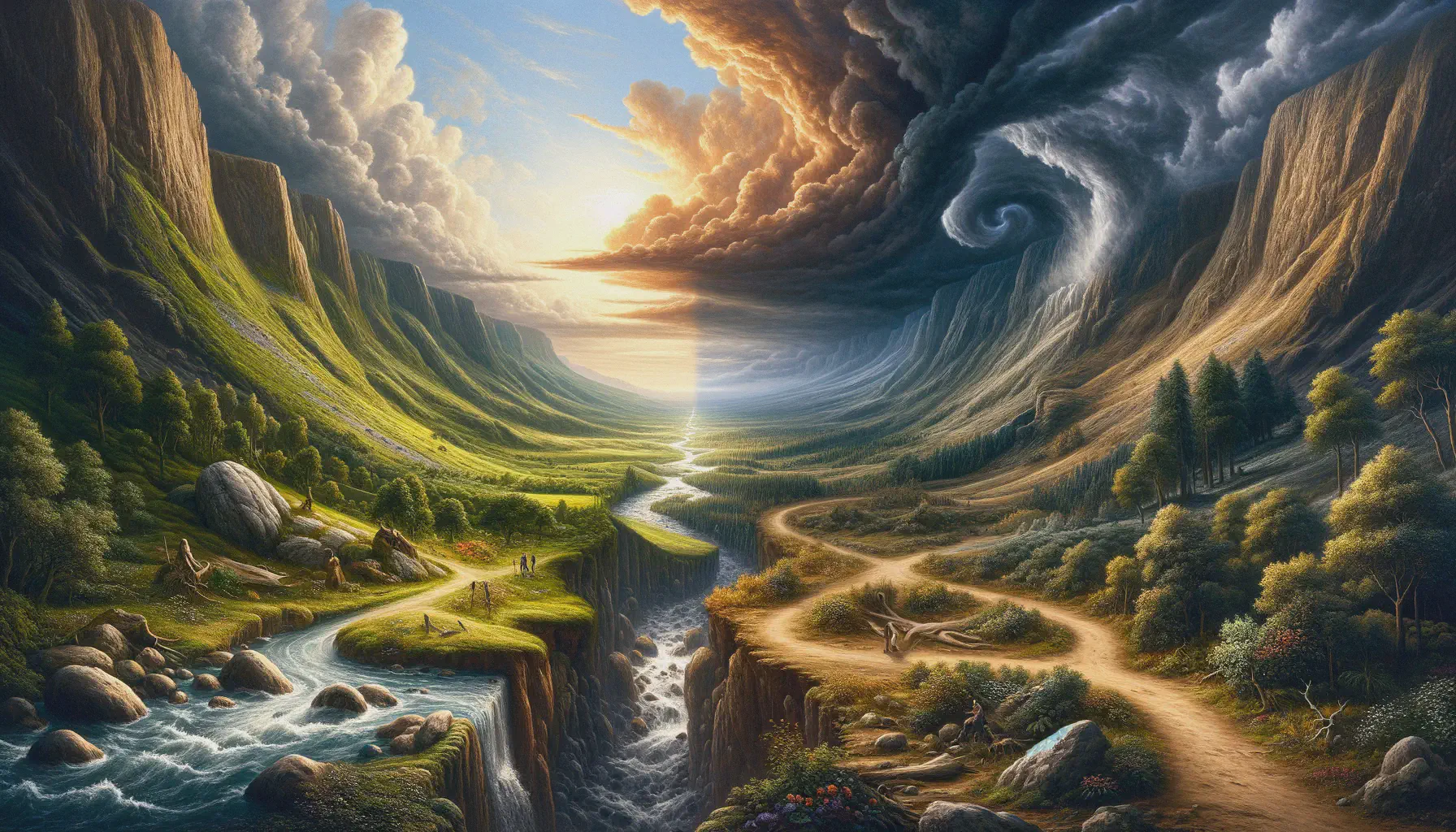
The two quantities we have been modelling (the time-dependent average and standard deviation of the returns) represent respectively the (potential) risk and reward associated with an asset. The relationship between these two quantities is implicit in the GARCH model. However, sometimes the return depends directly on the risk. A variant of the GARCH model can take this explicit relationship into account.
Reference Model
Let’s start by modelling the ACC returns using a GJR GARCH model with a Skewed Student-t Distribution.
specification <- ugarchspec(
distribution.model = "sstd",
mean.model = list(armaOrder = c(0, 0)),
variance.model = list(model = "gjrGARCH")
)
fit <- ugarchfit(data = ACC, spec = specification)
coef(fit)
mu omega alpha1 beta1 gamma1 skew
2.939585e-04 9.298925e-06 7.510285e-03 9.290941e-01 7.535600e-02 1.069799e+00
shape
6.093299e+00
The specification of mean.model
implies a time-independent average return.
GARCH-in-Mean Model
The GARCH-in-mean (GARCH-M) model integrates a risk term into the equation for the average return:
$$ \mu_t = \mu + \lambda\sigma_t. $$
If \(\lambda > 0\)
then higher returns are associated with greater risk and vice versa. π¨ We are no longer assuming a constant mean. In this model the mean changes with time.
To convert the reference model above into a GARCH-in-mean model we need to add some parameters to the mean.model
argument:
archm
β whether to include volatility in the mean; andarchpow
β the exponent of\(\sigma_t\)
.
specification <- ugarchspec(
distribution.model = "sstd",
mean.model = list(armaOrder = c(0, 0), archm = TRUE, archpow = 1),
variance.model = list(model = "gjrGARCH")
)
fit <- ugarchfit(data = ACC, spec = specification)
coef(fit)
mu archm omega alpha1 beta1
-3.240077e-03 2.117885e-01 1.125499e-05 6.353074e-03 9.217580e-01
gamma1 skew shape
7.913028e-02 1.071063e+00 6.019235e+00
The value of \(\lambda\)
is given by archm
.
An alternative formulation of the model sets
$$ \mu_t = \mu + \lambda\sigma_t^2. $$
specification <- ugarchspec(
distribution.model = "sstd",
mean.model = list(armaOrder = c(0, 0), archm = TRUE, archpow = 2),
variance.model = list(model = "gjrGARCH")
)
fit <- ugarchfit(data = ACC, spec = specification)
coef(fit)
mu archm omega alpha1 beta1
-0.0012773434 5.4817434401 0.0000114815 0.0060710444 0.9208525678
gamma1 skew shape
0.0798243808 1.0697491862 6.0407995156
AR(1) Model
The GARCH-in-mean model explicitly creates a dependency between risk and reward. An alternative approach is to use a model based on the correlation between successive returns. The AR(1) model looks like this:
$$ \mu_t = \mu + \rho\mu_{t-1}. $$
The behaviour of this model depends on the sign and magnitude of \(\rho\)
:
\(\rho > 0\)
β positive autocorrelation; if previous value above (below) long term mean then current value will be above (below) mean too (indicates under-reaction);\(\rho < 0\)
β negative autocorrelation; if previous value above (below) long term mean then current value will be below (above) mean (indicates over-reaction);\(|\rho| < 1\)
β mean reversion; after a shock the values decay to the mean;\(|\rho| ~ 1\)
β momentum; effects of a shock are more persistent.
If AR coefficient is > 0: “MOMENTUM” higher/lower average return followed by higher/lower average return. Market under-reacts, so still reacting next day. If |AR| coefficient is < 1: mean reversion If AR coefficient is < 0: “REVERSION” higher/lower average return followed by lower/higher average return. Market over-reacts, so correcting next day.
Create an AR(1) GJR GARCH model.
specification <- ugarchspec(
distribution.model = "sstd",
mean.model = list(armaOrder = c(1, 0)),
variance.model = list(model = "gjrGARCH")
)
fit <- ugarchfit(data = TATASTEEL, spec = specification)
ar1_mean <- fitted(fit)
ar1_vol <- sigma(fit)
coef(fit)
mu ar1 omega alpha1 beta1
1.310861e-03 -3.064634e-02 7.481843e-06 1.054478e-02 9.516358e-01
gamma1 skew shape
5.655076e-02 1.050852e+00 5.050698e+00
The negative value for ar1
implies that successive returns are anti-correlated: an above-average return is followed by a below-average return and vice versa.
This has positive ar1
. What does this mean?
fit <- ugarchfit(data = HDFC, spec = specification)
coef(fit)
mu ar1 omega alpha1 beta1 gamma1
6.018919e-04 1.797143e-02 7.484922e-06 2.516759e-02 9.108610e-01 7.901444e-02
skew shape
1.031812e+00 5.969515e+00
This has positive ar1
.
MA(1) Model
specification <- ugarchspec(
distribution.model = "sstd",
mean.model = list(armaOrder = c(0, 1)),
variance.model = list(model = "gjrGARCH")
)
fit <- ugarchfit(data = TATASTEEL, spec = specification)
coef(fit)
mu ma1 omega alpha1 beta1
1.311620e-03 -3.259906e-02 7.480174e-06 1.054758e-02 9.516881e-01
gamma1 skew shape
5.644991e-02 1.050552e+00 5.043684e+00
ARMA(1, 1) Model
specification <- ugarchspec(
distribution.model = "sstd",
mean.model = list(armaOrder = c(1, 1)),
variance.model = list(model = "gjrGARCH")
)
fit <- ugarchfit(data = TATASTEEL, spec = specification)
coef(fit)
mu ar1 ma1 omega alpha1
1.336495e-03 4.934020e-01 -5.313694e-01 7.294276e-06 1.124307e-02
beta1 gamma1 skew shape
9.519920e-01 5.503656e-02 1.046955e+00 4.989622e+00
Alternative Implementation
HOW IS THIS DONE WITH THE TSGARCH PACKAGE?
Letβs try with the {tsgarch}
package.
library(tsgarch)
Model Complexity
As we proceeded from a GARCH-in-Mean model to AR(1), MA(1) and ARMA(1, 1) models the number of parameters increased. This means that the models became progressively more complicated (and flexible). In general one should strive for a parsimonius model: just complicated enough to get the job done.